Copyright IBM Corp, 2008, 2010.Change history
How to Call AS/400 RPG Program from Java. Admin 21 February 2015 Java, Others. IBM has provided library for communicating with the AS/400 server from Java. The IBM Toolbox for Java is a library of Java classes that give Java programs easy access to IBM iSeries data and resources. We have two options to call the AS400 program from our Java. Calling Java methods from RPGLE programs. This post presents two IBM iSeries programs, a CLP and its associated RPG-LE program and a Java class. Together, they serve to illustrate how to call Java methods from an RPG program. The Class-methods reside in a.JAR-file deployed on the IFS. The CL Program. RPGLE Service Program Example - iSeries (AS400) Java convert hex string to byte array example; Java convert file data to String example. You may already know how to call a java class using RUNJVA or JAVA from iSeries. I am going show you a sample RPGLE code to call the java main() method. Just remember if you want a result back from the.
Common problems:
Frequently asked questions:
The call to a Java method is failing with RNX0301
- The Java exception is some other exception. Please see the section on failed Java method calls.
The Java method failed with a Java exception
To see the Java exception causing the error, inspect the RNX0301 message thatyou received. It should list the Java exception in the help for the message.
If the Java exception is one of these messages, please check that section ofthis document first: NoClassDefFound, orNoSuchMethodError.
If you want to see exactly which Java class threw the exception, see thesection on getting an exception trace from Java.
If you think the exception should not be occurring, check whether one of thefollowing problems could be causing the error:
- The Java class is version-sensitive, and you are not using the correctJava version at runtime. Please see the section on setting the Java versionat runtime.
- There is more than one version of the class in your classpath, and the wrongversion of the class is being used. Please see the section on determineexactly which Java classes are being used.
My Java class works fine when called from Java, but doesn'twork when called from RPG.
The most common cause of this problem is that the JVM is running under a differentJava version when you call the Java method from your RPG program.Please see the section on setting the Java version.
The application runs fine and then suddenly starts failing.
When you create Java objects from your RPG programs, or when you call Java methods thatreturn Java objects, the JVM keeps track of the fact that you have references to thoseobjects. Until you tell the JVM that you don't need those objects any more, it willprevent those objects from being freed during garbage collection. Eventually, itwill be unable to create any more objects.
Ensure you are freeing objects if you no longer need them. See the sectionon freeing objects.
There may be a Java system property that controls the number of objects that canbe created.Consult the list of the available Java system properties in the Javasection of the Information Center.
How can I set the Java version?
The Java version is set by the java.version system property.Please see the section on setting Java properties.
Why does Java seem to ignore myCLASSPATH or QIBM_RPG_JAVA_PROPERTIES environment variables?
- The environment variables are only used when the RPG runtime is starting the JVM. If theJVM has already been started, the environment variables would be ignored. The JVM may havealready been started; possibly by some other RPG program making a Java method call, orby some explicit JNI coding to start the JVM.
Tip: If you have several different RPG applications that all need to call Java methods,set up LEVEL(*SYS) environment variables for CLASSPATH and QIBM_RPG_JAVA_PROPERTIES; the classpathshould include all the classes that might be need by any application. With a LEVEL(*SYS) environmentvariable, every job starts with that environment variable already set. - The environment variables are case-sensitive. For example, an environment variablenamed 'classpath' or 'qibm_rpg_java_properties' would not be found.
- If the properties are not specified correctly, they will be ignored.Please the section on
Java properties.
How do I free my Java objects?
See the Java section in the ILE RPG Programmer's Guide information on how to free Javaobjects. You can free them one at a time, or free several objects at once.
If you are already freeing your objects, you may need to free them more often. Forexample, if you free all your objects at the end of your program, but within yourprogram you create several objects for each record of a file, then you should freeobjects after the processing for each record, rather than waiting until the end ofthe program.
How can I get an exception trace from Java?
To be able to use this support, you must also recompile your RPG program on a V5R3 or greater system, and the target release cannot be for a release earlier than V5R3.
Unlike other environment variables associated with Java, the QIBM_RPG_EXCP_TRACE environment variable may be set and unset at any time. It is checked whenever a Java method call ends with an exception.
int getTeacher(byte course[])
in class Student, then you would write the following Java class, and change a debug version of your RPG program to call method
byte[] debugGetTeacher(Student s, byte course[]) in class DebugStudent. The wrapper method will handle printing the exception trace.
How can I see the System.out and System.err output?
- If you run your program in batch, you will get a QPRINT file containing theJava output.
- If you run your program from QSH, you will get the output to the QSH screen. Torun your program from QSH, call it like this:Warning: If you call your program this way, be aware that the parameters will bepassed as null-terminated strings. In general, this way is only convenient ifyou have no parameters, since you would have to have different processing in yourprogram for your parameters, depending on whether you were called from the commandline or from QSH.
- Otherwise, you must do a few things to get the output. Note that the environmentvariables must be set before the JVM is started, and the file descriptors must beopened before any Java output is done:
- Set the Java property os400.stdout=file:path and os400.stderr=filepath, specifying the locations where you want the output to go. For example, if you use QIBM_RPG_JAVA_PROPERTIES to set the Java properties, and you want the output to go to files /mydir/out.txt and /mydir/err.txt, you would specify
- Set the environment variable QIBM_USE_DESCRIPTOR_STDIO to the value 'Y'.
- Check that the three standard-I/O file descriptors are open. Create the CHECKSTDIO program (source code below) and call it before you call your RPG application, or you could call it from your RPG application.
How do I set the class path?
The class path can be set by the CLASSPATH environment variable, or by thejava.class.path property. The class path consists of directories and jar files.The entries are separated by a colon.
For example, if you have .class objects in directory /mydir/myclasses and youalso have jar files /sysclasses/app1.jar and /myclasses/myjar.jar, thenyour classpath would look like this:
If you have a directory containing jar files, it is not sufficient tojust specify the directory. You must explicitly specify each jar file.
Also, see the section Why does Java seem to ignore myCLASSPATH or QIBM_RPG_JAVA_PROPERTIES environment variables.
How do I set Java properties?
You can set Java properties using the SystemDefault.properties file. There areseveral different places you can store this file; please see the Java documentationin the Info Center for more information.
You can set Java properties using the QIBM_RPG_JAVA_PROPERTIESenvironment variable.
If you use both the SystemDefault.properties file and the QIBM_RPG_JAVA_PROPERTIESenvironment variable, the properties specified by the environment variable willoverride the properties specified by the file.
Note: The Java properties are case-sensitive.For example, setting the 'JAVA.VERSION' property will have no effect; you must set the'java.version' property.
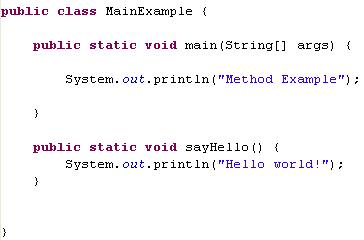
Note:Prior to V5R3, any setting for the java.version property in theSystemDefault.properties file was ignored when the JVM was started by the JNI (Java Native Interface).If you are on a release prior to V5R3, you will have to usethe QIBM_RPG_JAVA_PROPERTIES environment variable to setthis particular property.
How do I use the QIBM_RPG_JAVA_PROPERTIES environment variable?
The environment variable must be set before the JVM is created. The JVM will be createdwhen the first RPG program in the job calls a Java method. Any further changes to the environmentvariable will be ignored in that job.
The properties are specified the same as for the QSH java command, in the form '-Dproperty=value'.
The properties are case sensitive; 'java.version' is not the same as 'JAVA.VERSION'.
The value of the environment variable is in the form 'property;property;property;' whereeach property is terminated by a semicolon. (It is not strictly necessary to use a semicolon;other characters could be used as long as they are all the same, and as long as they are notused anywhere else in the actual properties.)
Examples:
For more information, please see the ILE RPG Programmer's Guide. The information is in all versions of themanual starting with the V5R3 manual.
Howcan I find out what Java version and classpath are being used in the JVM?
You can use the DMPJVM command to get information on the version and classpath andmany other things. The JVM must have already been started before DMPJVM is done.
Also, the DMPJVM command must be issued from a different job.An easy way to do that is tosubmit the DMPJVM command for your current job. For example, if your current job isQPADEV0002 JSMITH 023816, then you could do the following command, and then inspectthe spooled output.
You can also retrieve any system property by calling the getProperty method in theSystem class. See the source below,for a program that interactively displays properties.
Determine exactly which Java classes are being used at runtime
Specify the os400.run.verbose=true Java property.
Please see the section on setting Java properties for more information.
If the output flashes on the screen too fast for you to see it, or does not appear at all,please see the section on seeing the System.out and System.err output.
Changing Java classes after the JVM has been started
If the JVM has already been created in your job, and you thenchange a Java class, or create a new Java class, the changes will not berecognized by Java in that job. You must sign off and signback on again to have Java 'see' your changes.
While developing Java classes for use by RPG, it is sometimes more convenientto submit your tests to batch rather than continually sign off and back on.If you use SBMJOB for your tests, be sure to ask for your current environment variables tobe used for the submitted job:
Note: It is not necessary to start a new job if you only change your RPG programs.
The Java exception is NoClassDefFound
Iseries Java Version
If the class mentioned in the Java exception is not the same as the class of the methodbeing called, it is likely that the called Java method received thisexception. Please see the section on failed Java method calls.
The call could not complete because Java could not find the class specified by theRPG prototype for the Java call. Check the following. If you find any problems,correct them and try again.
- The EXTPROC for the Java method has the Java class spelled correctly, and with the correct case ('MYCLASS' and 'myclass' are not considered the same by Java).
- The class is in a jar file, but the jar file is not mentioned in the classpath. Please see the section on setting the classpath.
- The class is new on the system, and the JVM was previously started in the job (probably by other calls from RPG to Java). Please see the section on changing Java classes after the JVM has been started.
The Java exception is NoSuchMethodError

Note:If the class mentioned in the Java exception is not the same as the class of the methodbeing called, it is likely that the called Java method received thisexception. Please see the section on failed Java method calls.
If the class mentioned in the Java exception is the same as the classof the method being called, then the call could not completebecause Java could not find the method specified by theRPG prototype for the Java call.Check the following:
- The EXTPROC for the Java method has the Java class and method name spelled correctly, and with the correct case ('MYCLASS' and 'myclass' are not considered the same by Java).
- The Java method has the static keyword, but the STATIC keyword was not specified for the RPG prototype.
- The Java method has no return type, and the name of the method is the same as the name of the class. If this is the case, then the Java method is a constructor, and the special method 'name' of *CONSTRUCTOR must be used for the RPG prototype. Please read the section in the ILE RPG Programmer's Guide on how to code the RPG prototype for a Java constructor.
- The signature does not match the actual Java class. If this is the case, please read the section in the ILE RPG Programmer's Guide on how to code the RPG prototype for a Java method. To see the expected signature for the Java method, use the javap command; there is an example in the help for the RNX0301 message.
- The method is new in the class, or the signature (return type and parameters) have changed, and the JVM was previously started in the job (probably by other calls from RPG to Java). Please see the section on changing Java classes after the JVM has been started.
- The Java method requires a particular Java version. If this is the case, please see the sections on finding out what Java version is being used in the JVM and setting the Java version.
Source code for program CHECKSTDIO
This program can be used as part of the setup necessary to seethe Java output from an interactive job.See the section on How can I see the System.out and System.err output?for the other setup that is required.
This program should be called when the job starts, to ensure that the three 'Standard I/O'descriptors 0, 1, and 2 are opened.
Program CHECKSTDIO
Source code for program JAVAPROP
This program can be used for casual inspection of the Java propertiesin effect. Use the DMPJVM command if you wantfull information about the JVM.
Warnings:
- Running this program will start the JVM if it hasnot already started.If youhave some special code that runs before the JVM is started (forexample, to set up environment variables) be sure todo that first.
- This program assumes that you have a binding directory calledJNIUTIL containing the procedures getJniEnv, beginObjGroup and endObjGroup,and that the prototypes for these procedures are in a source file*LIBL/QRPGLESRC(JNIUTIL).You can find the source code for these procedures in the ILE RPG Programmer'sGuide.
If you aren't sure what the property names are, you could use theALLJVAPROP program. See its source below.
Source code for program ALLJVAPROP
This program can be used to see all the possible Java properties youcould set. Note that the warnings listed for the JAVAPROP program also apply to this program.
Details of changes to this document
- Version 2, 2010-06-02
- The recommended ALLOCSTDIO program was flawed in that it just opened three random descriptors, and it allowed them to be closed again.
It has been replaced by the CHECKSTDIO program which first checks whether descriptors 0, 1, and 2 are already open, and it checks whether they are correctly opened for input (0) or output (1 and 2).
The CHECKSTDIO program does not provide any way of closing the descriptors later.
- The recommended ALLOCSTDIO program was flawed in that it just opened three random descriptors, and it allowed them to be closed again.